SEGA Genesis: Building a ROM
This first article is focused on building a very simple ROM for the SEGA Genesis using assembly code. The ROM does a simple graphical effect, flickering the background color. Getting text on the screen is more complicated, so a proper 'Hello World' ROM will have to wait.
Genesis Hardware
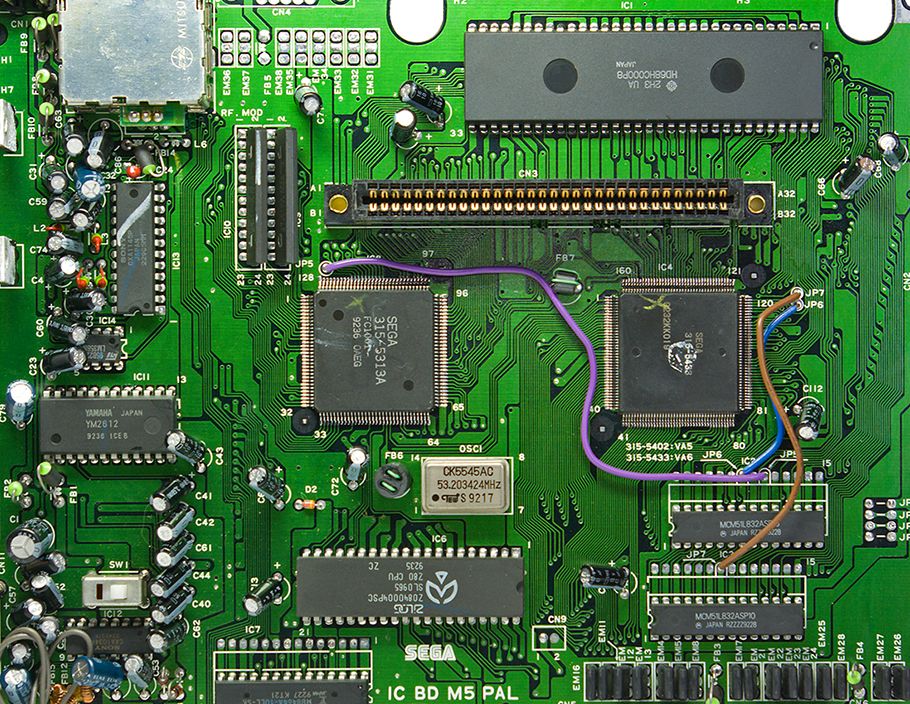
The SEGA Genesis had a Motorola 68000 CPU running at 7.6 MHz, a pretty fast processor for its time. It had very limited memory, only 64 KB main RAM and 64 KB Video RAM, half of what a decent home computer of the time would have, but that would be offset by the storage device being ROM cartridges with 512 KB - 8 MB, reducing any load time to almost zero.
The Genesis had a secondary CPU, a Zilog Z80 running at 3.58 MHz with 8 KB RAM. The Z80 was a very popular CPU in the late 70s and early 80s, used in the ZX Spectrum, the MSX computers, the Sega Master System, and in arcade games such as Pac-Man, Galaga, Dig Dug, Xevious, and others.
The Z80 was primarily used for controlling the audio chips, a Yamaha YM2612 FM chip which delivered 6 stereo FM channels and the Texas Instruments SN76489 Programmable Sound Generator (PSG), which has 3 square waves and a noise channel.
For this article, we're going to focus on the Motorola 68000.
The Motorola 68000
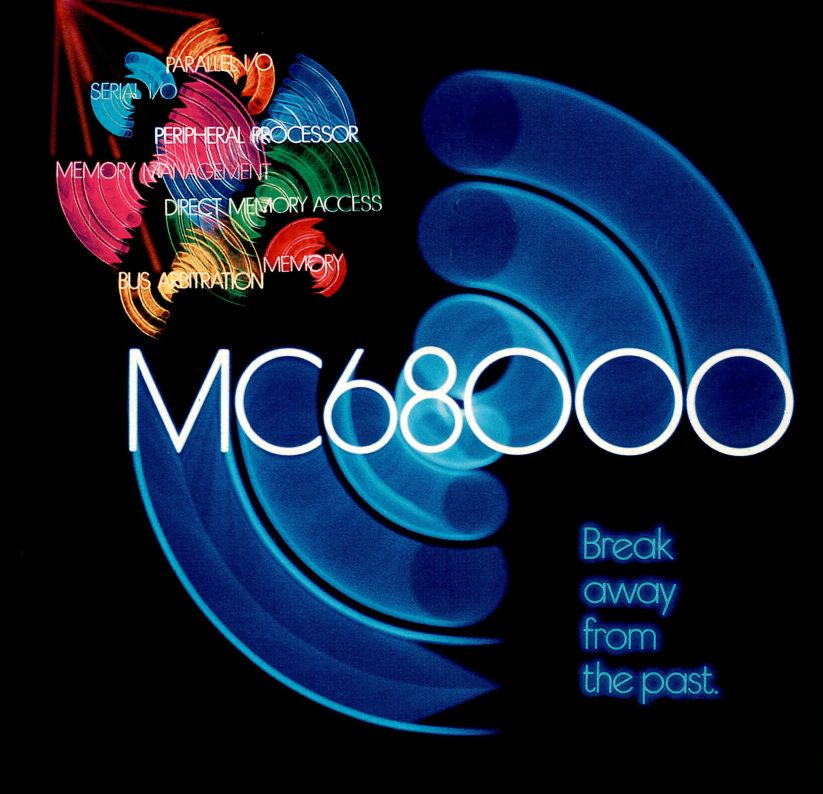
The Motorola 68000 is a 16/32-bit processor introduced in 1979. It was very popular in 80s computers, notably:
- The first Sun Microsystems workstation, Sun-1 (1982), the first generation of SunOS *nix workstations, funded by the Defense Advanced Research Projects Agency (DARPA).
- The Apple Lisa (1983) and the original Apple Macintosh (1984), the first Apple computers to have a graphical user interface.
- The Silicon Graphics IRIS 1000 (1984), a computer for professional graphics production, featuring a 1024x1024 resolution, and custom 3D rendering hardware.
- The Commodore Amiga (1985), a home computer known for its impressive graphics and 4-channel sampled sound, very popular in Europe.
- The Atari ST (1985), one of the most prominent computers used for home music production in the late 80s and early 90s.
- The Sharp X68000 (1987), notable for its great Capcom arcade ports.
It was also a popular CPU for arcade games:
- Sega's arcade hardware platform, System 16 (1985), known for Shinobi, Golden Axe, Altered Beast.
- Capcom's arcade system, CP System II (1993), known for Super Street Fighter II, the Street Fighter Alpha series, Darkstalkers, and Marvel vs. Capcom.
- SNK's 1990 arcade platform Neo Geo (1990), with games such as Metal Slug, Fatal Fury, King of Fighters, Samurai Shodown.
For the 1988 Genesis, the MC68000 was an obvious choice, it was already a mainstay in home computers, and SEGA already used it their System 16 arcade hardware, presumably making it much easier to port arcade games to the home market. In fact, the Genesis architecture is very similar to the System 16, which also features a Z80, a Yamaha FM chip, and a standard resolution of around 320x224.
Markey Jester's Motorola 68000 Beginner's Tutorial is useful for learning MC68000 assembly code, the game development language of choice for SEGA Genesis programmers.
If you want to test out some 68000 instructions or debug your code, EASy68K is really useful. It's an integrated development environment (IDE) that includes an editor, an assembler, and a debugger. It only emulates a 68000, no Genesis-specific stuff at all. It does have features for rendering text, graphics, and sound, so it can be a great test bed for certain types of problemsolving.
Tools
The only tool needed to create a ROM is an MC68000 assembler and a Z80 assembler. The open source assembler vasm works well for this purpose.
You should download these two builds:
We can test the MC68000 assembler with a simple program:
move.w #7,d0
A single instruction that sets register D0 to the value 7.
Assemble it using this command:
vasmm68k_mot -Fbin test.asm -o test.bin
The result is a 4 byte file test.bin
:
Offset(h) 00 01 02 03 04 05 06 07 08 09 0A 0B 0C 0D 0E 0F 00000000 30 3C 00 07 0<..
Generated by HxD